Reduce rendering time with large data in react
Introduction
Performance is key in modern web development. Managing efficient rendering becomes crucial as web applications grow in complexity and data volume. One effective technique for improving performance in React applications is virtualization. This article will explore virtualization, why it matters, and how to implement it in a React application.
What is Virtualization?
In web development, virtualization refers to rendering only the visible portion of a large data set to the DOM while keeping the rest in memory. This approach significantly reduces the number of DOM nodes, enhancing rendering performance and improving the overall user experience.
Problem Overview
When dealing with large lists or grids in React, rendering all components at once can lead to several performance issues. Here’s a breakdown of the common problems developers face:
- Excessive DOM Updates
React renders all components in a list by default, and if that list is large (for example, thousands of items), this results in a large number of DOM nodes being created. Such a large volume of DOM updates can cause performance bottlenecks, making the application sluggish. - Increased Memory Usage
Loading all the components in a large list at once can consume a significant amount of memory. Browsers may struggle to manage such a large number of DOM elements, resulting in crashes or unresponsive pages. - Long Initial Load Times
Without using virtualization, React renders the entire list or grid as soon as the component mounts, leading to longer load times. This can create a poor user experience, as users might see blank screens or experience delays in loading when they expect immediate interaction. - Unresponsive Scrolling
Rendering large lists all at once can cause the UI to become unresponsive during scrolling. Since React has to update the DOM for every scroll event, users may experience laggy or delayed scrolling behaviour, often referred to as “janky scrolling.”
How to Tackle Rendering Large Lists in React?
If you end up in a place where you have to render tens of thousands of complex list items, you would inevitably have to use one of the following solutions, no matter how capable your end user’s hardware is. Firstly we’ll start with a general solution that everyone can incorporate into their code:
Why Virtualization Matters: Trade-offs
While virtualization drastically improves performance, it’s important to understand the trade-offs:
- Initial Complexity: Implementing virtualization adds complexity to your codebase compared to a simple list rendering. However, tools like react-virtualized mitigate this with pre-built components.
- Scrolling Behavior: Virtualization renders only a subset of list items at a time, which can result in some items briefly disappearing during fast scrolling, depending on your settings.
- Overhead: Virtualization introduces some overhead by calculating which items are visible and managing off-screen data. Although this is minimal compared to rendering all elements, it’s worth considering.
Ultimately, the performance gains far outweigh these trade-offs for lists with over a few hundred items.
Performance Benchmark: Before and After Virtualization
Before –
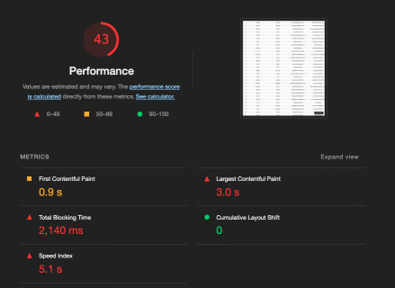
Lighthouse score
After adding virtualization-
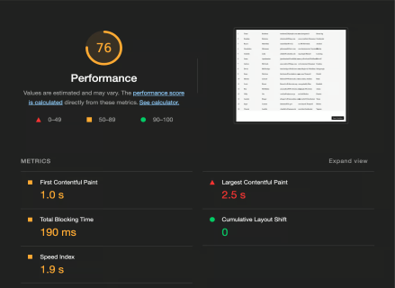
Lighthouse score of massive list with virtualization
Setting Up the Project
To demonstrate virtualization, we’ll create a simple React application using the react-virtualized library.
Step 1: Create a React Application
npx create-react-app react-virtualization-example cd react-virtualization-example npm install react-virtualized
Step 2: Basic Component Setup
Create a component to display a virtualized list.
// src/VirtualizedList.js import React from 'react'; import { List } from 'react-virtualized'; import 'react-virtualized/styles.css'; // only needs to be imported once const VirtualizedList = ({ items }) => { const rowRenderer = ({ key, index, style }) => ( <div key={key} style={style} className="list-item"> {items[index]} </div> ); return ( <List width={300} height={600} rowCount={items.length} rowHeight={50} rowRenderer={rowRenderer} /> ); }; export default VirtualizedList;
Integrating the Virtualized Component
Step 3: Use the Virtualized Component in Your App
// src/App.js import React from 'react'; import VirtualizedList from './VirtualizedList'; const App = () => { const items = Array.from({ length: 100000 }, (_, index) => `Item ${index + 1}`); return ( <div className="App"> <h1>Virtualized List Example</h1> <VirtualizedList items={items} /> </div> ); }; export default App;
Advanced Usage
Step 4: Customizing the Virtualized List
Explore advanced features of react-virtualized such as dynamic row heights, infinite scrolling, and more.
import React from 'react'; import { InfiniteLoader, List, AutoSizer } from 'react-virtualized'; const InfiniteVirtualizedList = ({ loadMoreRows, isRowLoaded, rowCount }) => { const rowRenderer = ({ key, index, style }) => ( <div key={key} style={style} className="list-item"> {`Item ${index + 1}`} </div> ); return ( <InfiniteLoader isRowLoaded={isRowLoaded} loadMoreRows={loadMoreRows} rowCount={rowCount} > {({ onRowsRendered, registerChild }) => ( <AutoSizer> {({ height, width }) => ( <List height={height} width={width} onRowsRendered={onRowsRendered} ref={registerChild} rowCount={rowCount} rowHeight={50} rowRenderer={rowRenderer} /> )} </AutoSizer> )} </InfiniteLoader> ); }; export default InfiniteVirtualizedList;
Conclusion
Virtualization is a powerful technique to enhance the performance of React applications dealing with large data sets. By rendering only the visible items, we can significantly improve the efficiency and responsiveness of our apps. Tools like react-virtualized make implementing this technique straightforward, providing a range of features to cater to various use cases.
Our React js competency understands the key features, toolchain, and React API libraries to build lightweight and interactive applications. Reach out to our experts to know more.