Handling Large File Uploads in React: The Multipart Upload Approach
In my last project, I ran into a problem: how to handle large file uploads in a React app. The single request file upload wasn’t working for files over 100MB. Network instability, slow speeds, and interruptions caused failed uploads and a bad user experience. That’s when I looked into multipart file uploads—a technique that involves splitting the file into smaller chunks and uploading one by one.
The Challenge
Users were uploading large video files and we needed a solution that could handle these uploads without failure. The main challenge was to make sure the file upload wouldn’t have to restart from scratch if something went wrong during the upload. Restarting an upload from scratch when a network issue occurs can be frustrating for users especially if the file is large. That’s where multipart uploads came to the rescue.
The Multipart Upload Approach
With multipart file uploads you split the file into smaller chunks (in our case 50MB each). These chunks are then uploaded individually to the server. Once all the parts are uploaded the server assembles them into a single file.
The process involves several steps:
- Initialize the multipart upload: The first step is to notify the server that an upload is starting which returns an upload session ID that tracks the entire process.
- Split the file into parts: The file is divided into smaller parts based on a predefined size limit.
- Get pre-signed URLs: For each file part, the server provides a pre-signed URL. This URL allows the client to upload each file chunk directly to cloud storage.
- Upload each part: Using the pre-signed URLs each part is uploaded to the server.
- Complete the upload: After all parts are uploaded the server is notified to assemble them into the final file.
Progress Tracking and User Feedback
One of the best things about this approach is that we can track progress. By monitoring each part upload we can calculate the total percentage of the file uploaded and show it to the user. This improves the user experience and gives the user an idea of whether the upload is on track or if something went wrong.
Cancelling the upload
We also implemented the ability to cancel the upload mid-way. So if the user changes their mind they don’t have to wait for the entire file to finish uploading before stopping the process. Using Axios’ CancelToken we were able to implement a simple cancel feature that stops all active file uploads instantly.
Conclusion
Multipart file uploads in React were a total game-changer for handling files of a larger size. We could split files into manageable pieces and upload them one by one. This made our uploads much more reliable and user-friendly. This approach enabled us to deliver file uploads resilient to interruptions; we were able to track the progress in real-time, and even offer an option to cancel the upload at any point.
Next time you are faced with the need to upload large files in your React application, do it using this multipart upload approach. It is a powerful method that saves time, improves user experience, and ensures reliable yet efficient uploads.
Code –
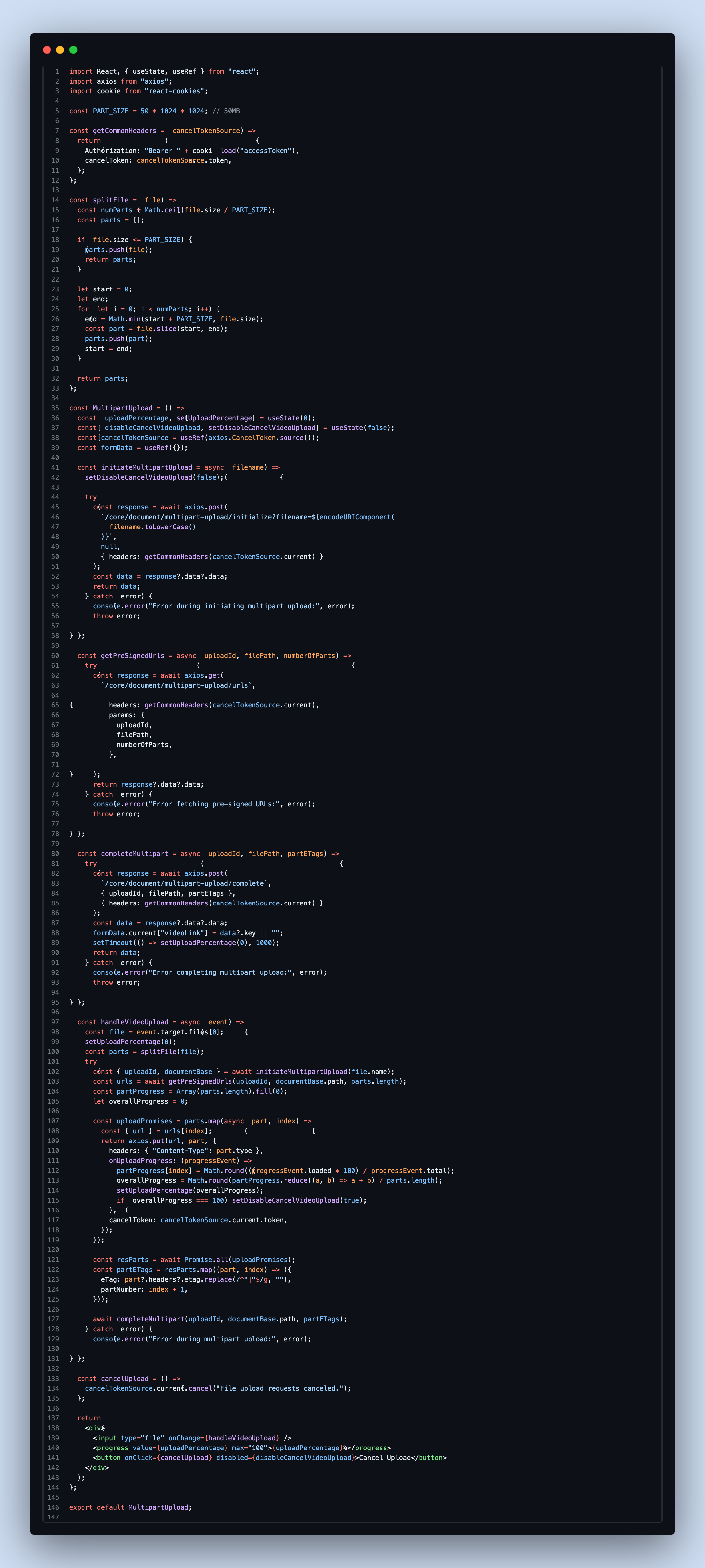
multipart file upload code