Design & Implement a Event-Driven Architecture in Python
Introduction
In today’s world of distributed systems, scalability, performance, and responsiveness are key requirements for many applications. One architectural pattern that helps achieve these goals is the Event-Driven Architecture (EDA). This architecture enables systems to be loosely coupled, scalable, and highly responsive to events. In this blog, we’ll explore what EDA is, why it’s beneficial, and how to design and implement a scalable event-driven system in Python.
1. What is Event-Driven Architecture?
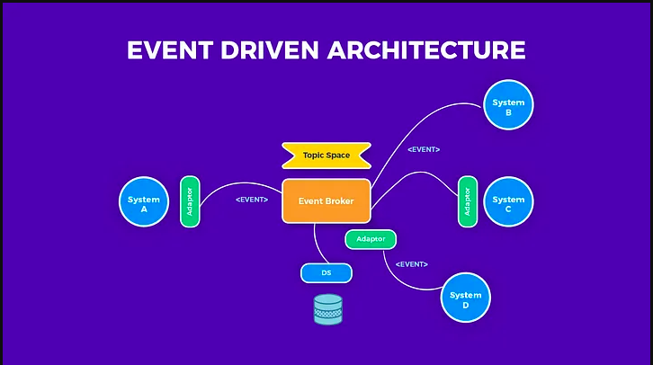
*
Event-driven architecture is a software design pattern where systems react to events-changes in state or user actions. These events trigger responses (known as event handlers or consumers) which execute predefined logic. The architecture revolves around three key components:
- Producers (Emitters): These are responsible for generating events.
- Events: These represent changes or actions that are broadcast to the system.
- Consumers (Listeners): These respond to events by executing corresponding business logic.
This pattern allows for loose coupling, where producers and consumers operate independently and communicate asynchronously, often using message brokers or event buses.
Key Benefits of EDA:
- Scalability: Systems can handle a large number of events asynchronously.
- Decoupling: Producers and consumers can evolve independently without tightly integrating with each other.
- Responsiveness: Consumers react to events immediately, making the system more responsive to user actions or system changes.
2. Why Use Event-Driven Architecture in Python?
Python’s versatility, combined with its rich ecosystem of libraries and frameworks, makes it an excellent choice for building event-driven systems. Whether you’re building real-time applications, microservices, or distributed systems, EDA helps ensure your Python application can scale efficiently.
Read More: PDF Utilities using Python
Here are a few reasons to adopt EDA in Python:
- Asynchronous Processing: Python’s `asyncio` and libraries like Celery allow for non-blocking event loops, enabling systems to handle thousands of events concurrently.
- Message Brokers Integration: Python has strong support for integrating with message brokers such as RabbitMQ, Kafka, and Redis Pub/Sub, enabling reliable communication between producers and consumers.
- Microservices Support: EDA works well with microservices, and Python’s lightweight frameworks (Flask, FastAPI) are ideal for developing event-driven microservices.
3. Designing an Event-Driven Architecture
When designing a scalable event-driven system, it’s crucial to plan how the components (producers, events, consumers) will interact and scale.
Here’s a step-by-step guide:
3.1. Event Flow Design
The first step is defining how events flow through the system:
-
-
- Producers generate events: These could be user actions (e.g., clicking a button) or system-generated events (e.g., a file upload completion).
- Event broker transports events: A message broker (RabbitMQ, Kafka, etc.) handles event transport from producers to consumers.
- Consumers process events: Consumers receive events and trigger the required actions or responses.
-
3.2. Event Broker
The event broker is central to EDA. It enables asynchronous communication between services, allowing them to scale independently. Popular brokers for Python include:
-
-
- RabbitMQ: A message broker that supports complex routing, topic exchanges, and message durability.
- Apache Kafka: A distributed event-streaming platform that handles large volumes of data with high throughput and fault tolerance.
- Redis Pub/Sub: Lightweight and fast, Redis is ideal for real-time event-driven applications.
-
3.3. Asynchronous Processing
Python’s built-in `asyncio` module, as well as external tools like Celery and Dramatiq, enable non-blocking, asynchronous task execution. These tools ensure that event consumers can process tasks concurrently, preventing bottlenecks.
3.4. Event Types and Payloads
Design event types carefully, ensuring that events carry the necessary information in their payloads:
-
-
- Simple events: Carry minimal data, such as user IDs or order IDs, with consumers fetching additional data.
- Rich events: Carry all necessary data, such as a complete order object, allowing consumers to process them without further lookups.
-
4. Implementing Event-Driven Architecture in Python
Let’s implement a basic event-driven system in Python using RabbitMQ and Celery to handle events asynchronously.
Read More: Managing Dead Letter Queues(DLQ) in RabbitMQ with Java Spring Boot
4.1. Setting Up RabbitMQ
First, install RabbitMQ on your machine. It will act as the message broker between the producer and consumers.

*
4.2. Setting Up Celery for Event Handling
Install Celery and RabbitMQ’s Python client library.

*
Next, create a Celery instance for handling tasks (event consumers) asynchronously.
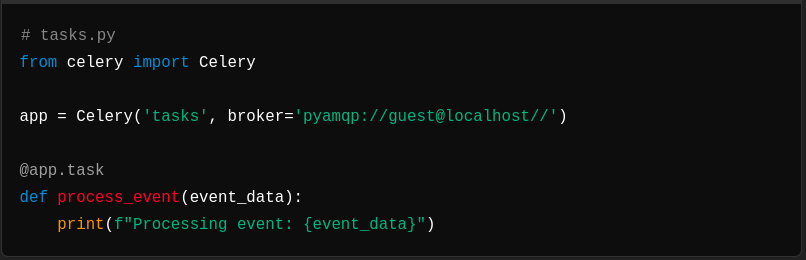
*
4.3. Producer (Event Emitter)
Producers will emit events to RabbitMQ, which Celery will consume.
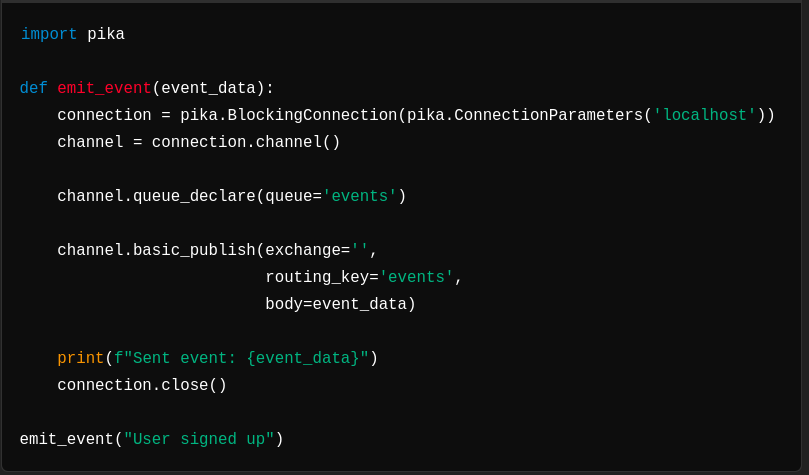
*
4.4. Consumer (Event Handler)
Finally, we run the Celery worker to consume events from RabbitMQ.

*This will start the Celery worker, which listens to RabbitMQ and processes events using the `process_event` task.
4.5. Scaling Consumers
To scale consumers, simply start more Celery workers.
RabbitMQ will distribute events across the workers, allowing your system to handle a large volume of events in parallel.

*This command runs 4 workers concurrently, enabling your system to process multiple events simultaneously.
5. Challenges in Scaling Event-Driven Architectures
While EDA offers many benefits, it comes with certain challenges, especially at scale:
- Event Duplication: Sometimes, events may be duplicated due to retries or broker issues. Consumers need to handle duplicate events idempotently.
- Order of Events: In distributed systems, ensuring the correct order of events is challenging. Solutions like Kafka’s partitioning can help maintain order.
- Error Handling: You need robust error handling and retries in case events fail to process.
Conclusion
Designing and implementing a scalable Event-Driven Architecture in Python allows you to build responsive, efficient, and loosely coupled systems. By leveraging Python’s rich ecosystem of asynchronous tools and message brokers like RabbitMQ or Kafka, you can build systems that handle large-scale, real-time event processing effectively.
EDA is particularly useful in microservices architectures, real-time applications, and systems that require scalability. While it comes with certain challenges, careful planning and design can mitigate these issues, making EDA a powerful pattern for modern Python applications.
Whether you’re working on a distributed microservices system or a real-time application, adopting an event-driven and agile approach can significantly improve your system’s scalability and resilience. So, consider EDA in your next Python or digital engineering project to handle events in a more efficient and scalable way!