Understanding State Batching in React
Introduction
In the world of React, efficient rendering and performance optimization are the keys to success. One of the most important concepts that plays a key role in React state updates is State Batching/Automatic Batching. This mechanism was introduced in React version 18, which significantly impacts the application’s performance and management of the state updates. In this blog, we’ll dive deep into the concept and break down all the mysteries of state batching, why it is important, and how we can manage it effectively in our React applications.
What is State Batching?
When we set a state variable in React, it triggers a re-render. However, there are times when we might want to perform several operations on the state value before initiating the next render. To manage this effectively, we play by the rules of the state batching mechanism. State batching is a performance optimization technique used by React to minimize the number of re-renders and improve the efficiency of updates. Instead of processing each state update immediately, React groups multiple state updates and processes them in a single render. This ensures that components are only re-rendered once, regardless of how many state updates are triggered.
Let’s break down state batching in the simplest terms:
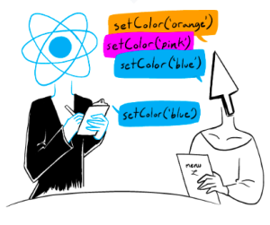
The Dinner Party Analogy
Imagine you’re hosting a dinner party and you have a rule that you’ll only serve food when you have a full tray.
Without Batching: Every time someone asks for a drink or a snack, you immediately go to the kitchen and bring it out, even if it’s just one item. So, you’re making many trips back and forth to the kitchen.
With Batching: Instead, you wait until several guests have requested different items. Once you have a full tray of requests, you go to the kitchen, fill the tray, and serve everything in one trip. This way, you make fewer trips and get everything delivered faster and more efficiently.
Also Read: Powering Dynamic Web Apps: Integrating React with Drupal 10 API for Security and Efficiency
Now let’s try to understand how this works using code:
import { useState } from 'react'; export default function Counter() { const [count, setCount] = useState(0); const handleClick = () => { setCount(count + 1); setCount(count + 1); setCount(count + 1); setCount(count + 1); setCount(count + 1); }; return ( <> <h1>{count}</h1> <button onClick={handleClick}>+5</button> </> ); }
Output:
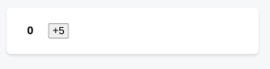
This image represents the initial render of the react application having an initial value of count (i.e. 0) and a button +5 render of the react application.
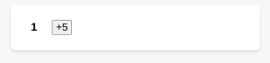
This image represents the incremented count value (i.e. 1) after clicking on the +5 button.
Explanation:
- According to the first look of the above code, as we click on the ‘+5’ button, it is expected to display ‘5’ as the output, due to setCount (count +1) being called 5 times in a row causing it to re-render 5 times. However, as the second image represents, it displays the count state as ‘1’ when the button is clicked.
- According to React, every state value being rendered is fixed. Therefore, the value of the state (count) inside the first render’s event handler is always 0. No matter how often it has been triggered, React will wait until all code in the event handlers has run before processing the state updates.
- This is why the re-render only happens after all the setCount() are called. This prevents us from re-rendering as we update multiple states being called from the same or different components without triggering re-renders. This concept is known as Batching where the React waits until all code in the event handlers has run and then starts updating the UI. This process helps React applications to run faster.
Why State Batching is important?
- Performance Optimization: By batching state updates, React reduces the number of re-renders and therefore the amount of work needed to update the DOM is decreased which leads to smoother and more efficient applications.
- Predictable Behavior: With state batching, state updates are predictable and less prone to causing race conditions or unexpected behaviors as React processes all state updates in a controlled manner.
Updating the same state multiple times before the next render
There can be an uncommon scenario where we would like to update the same state variable multiple times before the next render happens. To handle this kind of case, instead of passing the next state like setCount(count+1), we can pass a function that calculates the next state based on the previous one, like setCount(prevCount => prevCount + 1). Using this method we are telling React to remember the previous state and perform the task, instead of replacing it.
So, if we replace setCount(count+1) to setCount(prevCount => prevCount + 1) then prevCount => prevCount => prevCount + 1 will act as an updater function which will queue the previous value for further tasks.
Queued Update | prevCount | returns |
prevCount + 1 | 0 | 0 + 1 = 1 |
prevCount + 1 | 1 | 1 + 1 = 2 |
prevCount + 1 | 2 | 2 + 1 = 3 |
prevCount + 1 | 3 | 3 + 1 = 4 |
prevCount + 1 | 4 | 4 + 1 = 5 |
So, when we call the updater function, it gets added to a queue. When React calls useState for the next render, it goes through the queue. In our case, the initial value of “count” (i.e. 0) will be passed as an argument to prevCount in the updater function. React takes the returned value of our previous updater function and passes it to the next updater as prevCount and so on, storing ‘5’ as the final result. That is how we can update the state using the previous state value.
Conclusion
State Batching is a crucial optimization in React, that enhances performance and consistency by grouping multiple state updates into a single render pass. Understanding how State Batching works can help one write more efficient and predictable React applications. By leveraging State Batching effectively and keeping up with React’s advancements, we can build smoother and more responsive user interfaces that provide a better experience for all users. Get a free consultation on how we can help with Web Development services. Click here to schedule a meeting with us.